
Topic: Functions
Program requirements:
Displays your first and last name
Notes:
Use a function to display your first name
Use a function to display your last name
Use the println macro to display messages to the terminal
function to display your first name
fn first_name() -> String { "mart".to_string()
} // function to display your last name
fn last_name() -> String { "ola".to_string()
} fn main() { println!("my name is {} {}", first_name(), last_name());
}
Topic: Basic arithmetic
Program requirements:
Displays the result of the sum of two numbers
Notes:
Use a function to add two numbers together
Use a function to display the result
Use the “{:?}” token in the println macro to display the result
fn add(a: f64, b: f64) -> f64 { a + b
} fn main() { let result = add(1.0, 2.0); println!("The result is: {:?}", result);
}
Topic: Flow control using if..else
Program requirements:
Displays a message based on the value of a boolean variable
When the variable is set to true, display “hello”
When the variable is set to false, display “goodbye”
Notes:
Use a variable set to either true or false
Use an if..else block to determine which message to display
Use the println macro to display messages to the terminal
fn main() { let fact = true; // * Use a variable set to either true or false // * Use an if..else block to determine which message to display // * Use the println macro to display messages to the terminal if fact { println!("hello"); } else { println!("goodbye"); }
}
Topic: Flow control using if..else if..else
Program requirements:
Display “>5”, “<5”, or “=5” based on the value of a variable
is > 5, < 5, or == 5, respectively
Notes:
Use a variable set to any integer value
Use an if..else if..else block to determine which message to display
Use the println macro to display messages to the terminal
fn main() { let compare = 5; if compare > 5 { println!("{} is > 5", compare); } else if compare < 5 { println!("{} is < 5", compare); } else { println!("{} is = 5", compare); }
}
Topic: Decision making with match
Program requirements:
Display “it’s true” or “it’s false” based on the value of a variable
Notes:
Use a variable set to either true or false
Use a match expression to determine which message to display
fn main() { let set = true; match set { true => println!("it's true"), false => println!("it's false"), }
}
Topic: Decision making with match
Program requirements:
Display “one”, “two”, “three”, or “other” based on whether
the value of a variable is 1, 2, 3, or some other number,
respectively
Notes:
Use a variable set to any integer
Use a match expression to determine which message to display
Use an underscore (_) to match on any value
fn main() { let number = 100; match number { 1 => println!("one"), 2 => println!("two"), 3 => println!("three"), _ => println!("other"), }
}
Topic: Looping using the loop statement
Program requirements:
Display “1” through “4” in the terminal
Notes:
Use a mutable integer variable
Use a loop statement
Print the variable within the loop statement
Use break to exit the loop
fn main() { let mut iter = 1; loop { println!("{}", iter + 1); iter += 1; if iter == 4 { break; } }
}
Topic: Looping using the while statement
Program requirements:
Counts down from 5 to 1, displays the countdown
in the terminal, then prints “done!” when complete.
Notes:
Use a mutable integer variable
Use a while statement
Print the variable within the while loop
Do not use break to exit the loop
fn main() { let mut countdown = 5; while countdown > 0 { println!("{}", countdown); countdown -= 1; }
}
Topic: Working with an enum
Program requirements:
Prints the name of a color to the terminal
Notes:
Use an enum with color names as variants
Use a function to print the color name
The function must use the enum as a parameter
Use a match expression to determine which color
name to print
enum Color { Red, Pink, White,
} fn pintcolor(color: Color) { match color { Color::Red => println!("Red"), Color::Pink => println!("pink"), Color::White => println!("white"), }
} fn main() { pintcolor(Color::White)
}
Topic: Organizing similar data using structs
Requirements:
Print the flavor of a drink and it’s fluid ounces
Notes:
Use an enum to create different flavors of drinks
Use a struct to store drink flavor and fluid ounce information
Use a function to print out the drink flavor and ounces
Use a match expression to print the drink flavor
enum Drinks { Orange, Coke, Fanta, Lime,
}
// * Use a struct to store drink flavor and fluid ounce information
struct DrinksFlavor { flavor: Drinks, fluid: f64,
}
// * Use a function to print out the drink flavor and ounces
fn print_drinks(flavor: DrinksFlavor) { // * Use a match expression to print the drink flavor match flavor.flavor { Drinks::Orange => println!("orange"), Drinks::Coke => println!("coke"), Drinks::Fanta => println!("fanta"), Drinks::Lime => println!("lime"), } println!("{} ounces", flavor.fluid);
} fn main() { let lime = DrinksFlavor { flavor: Drinks::Lime, fluid: 1.0, }; print_drinks(lime); let orange = DrinksFlavor { flavor: Drinks::Orange, fluid: 1.0, }; print_drinks(orange); let coke = DrinksFlavor { flavor: Drinks::Coke, fluid: 1.0, }; print_drinks(coke); let fanta = DrinksFlavor { flavor: Drinks::Fanta, fluid: 1.0, }; print_drinks(fanta);
}
Topic: Data management using tuples
Requirements:
Print whether the y-value of a cartesian coordinate is
greater than 5, less than 5, or equal to 5
Notes:
Use a function that returns a tuple
Destructure the return value into two variables
Use an if..else if..else block to determine what to print
Use a function that returns a tuple
#[derive(Debug)]
struct Quantity { grocery: Box<str>, quantity: i32, id: i32,
} fn display_quantity(grocery: &Quantity) { println!( "{:#?} {:?} {:?}", grocery.grocery, grocery.quantity, grocery.id );
} fn main() { let grocery = Quantity { grocery: "garri".to_string().into(), quantity: 1, id: 4, }; display_quantity(&grocery); display_quantity(&grocery); display_quantity(&grocery);
} 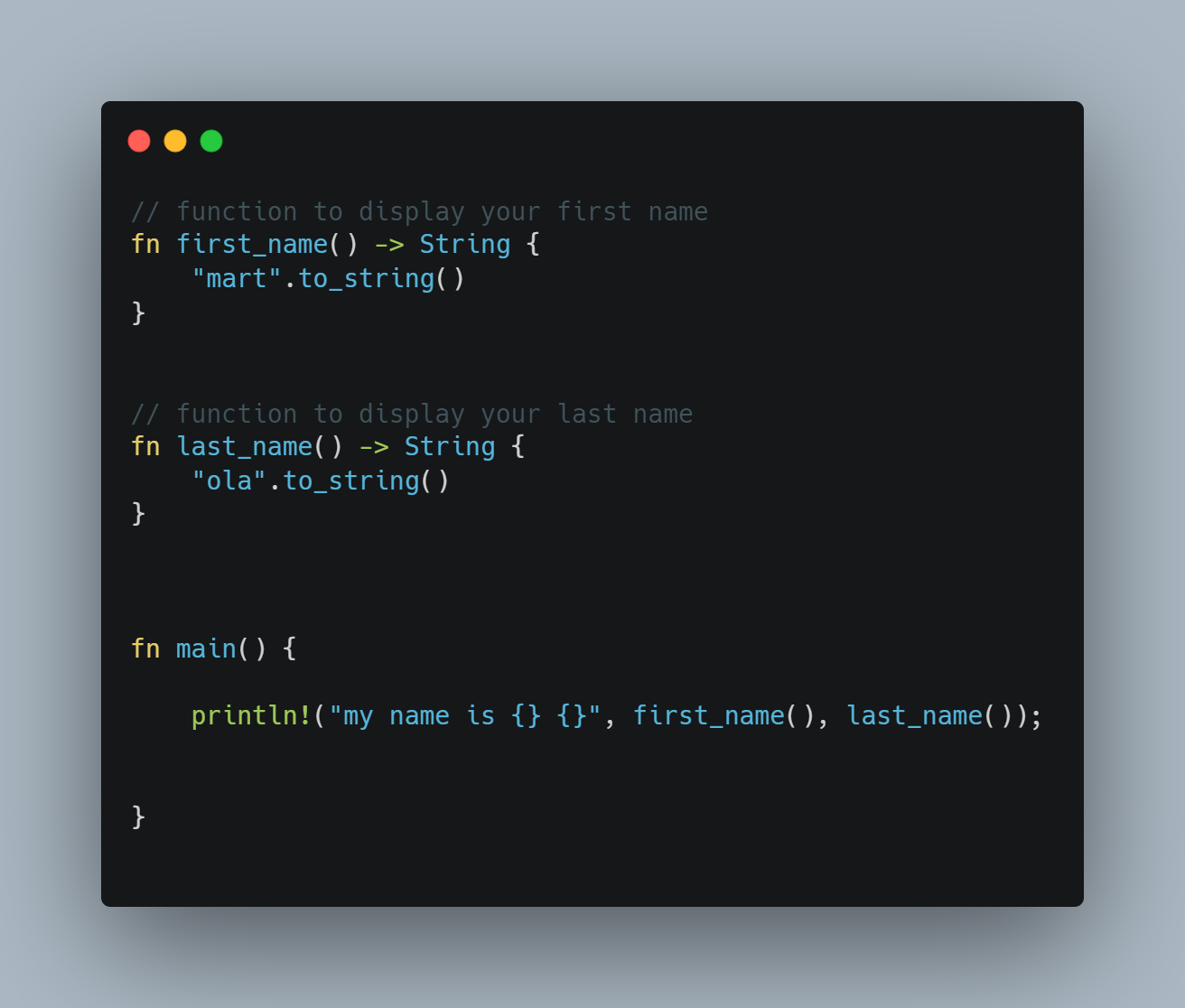
Discover more from Coursity
Subscribe to get the latest posts sent to your email.